Tuesday, December 22, 2015
Wednesday, December 16, 2015
Moving IIS Web Server folders to different drive.
I received new web servers that had very less disk space on c drive.If you install IIS web server,by default the web server folders i.e. inetpub will be located on the systemdrive,usually c drive. We had application logs that would require large space.In order to ensure IIS worked with folders on non system drive we had to do the following steps after installation
- Disable the Default Web Site in inetmgr
- Move the inetpub folder to D drive.You will require Admin Permissions on the server.
- Click on Default Web Site Basic Settings in inetmgr
- Point the physical path to the new folder on the non system drive.In this case it is D drive.
- Restart the Default Web Site in inetmgr
Friday, November 6, 2015
IIS - Automatically Restart stopped AppPools
I have applications that are hosted across 5 load balanced web servers with app pools for each site on the web servers. Recently I noticed that few App Pools had stopped and I had to log on to the server and restart the App pools.
Monday, October 5, 2015
c# - List all dates in a year that are a palindrome
I got a text message forward that today 5 October 2015, is a palindrome date. If you consider day first format (ddMMyyyy) then this would be 5102015.So I wanted to see if there are other dates in the year that are a palindrome.
In US the date format is always month first so for this program I am using the MMddyyyy format.So Sunday,May 10 2015 is a Palindrome.
using System;
namespace DatePalindrome
{
class Program
{
static void Main(string[] args)
{
try
{
DateTime oFirstDay = new DateTime(DateTime.Now.Year, 1, 1);
DateTime oLastDay = new DateTime(DateTime.Now.Year, 12, 31);
DateTime oDay = oFirstDay;
while (oDay < oLastDay.AddDays(1))
{
string sDay = oDay.ToString("MMddyyyy").StartsWith("0") ? oDay.ToString("MMddyyyy").Substring(1, 7) : oDay.ToString("MMddyyyy");
char [] sArray = sDay.ToCharArray();
Array.Reverse(sArray);
if (sDay.Equals(new string(sArray)))
Console.WriteLine("Palindrome:{0} - Day:{1}",sDay,oDay.ToLongDateString());
oDay = oDay.AddDays(1);
}
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
Console.WriteLine(ex.StackTrace);
}
Console.WriteLine("Done! Press any key to exit");
Console.Read();
}
}
}
In US the date format is always month first so for this program I am using the MMddyyyy format.So Sunday,May 10 2015 is a Palindrome.
using System;
namespace DatePalindrome
{
class Program
{
static void Main(string[] args)
{
try
{
DateTime oFirstDay = new DateTime(DateTime.Now.Year, 1, 1);
DateTime oLastDay = new DateTime(DateTime.Now.Year, 12, 31);
DateTime oDay = oFirstDay;
while (oDay < oLastDay.AddDays(1))
{
string sDay = oDay.ToString("MMddyyyy").StartsWith("0") ? oDay.ToString("MMddyyyy").Substring(1, 7) : oDay.ToString("MMddyyyy");
char [] sArray = sDay.ToCharArray();
Array.Reverse(sArray);
if (sDay.Equals(new string(sArray)))
Console.WriteLine("Palindrome:{0} - Day:{1}",sDay,oDay.ToLongDateString());
oDay = oDay.AddDays(1);
}
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
Console.WriteLine(ex.StackTrace);
}
Console.WriteLine("Done! Press any key to exit");
Console.Read();
}
}
}
sql - Side by side counts from multiple tables
I had to create a daily report with counts from different tables in SQL Server.The report had counts for total rows in tables for a given day.Below is the sql to get counts from 7 different tables and display the counts in separate columns for a given day in the month.
select a.create_dt,con,bro,[set],conf,demo,vip,dev
from
(select create_dt,count( id) con,row_number() over (order by create_dt) as row_num
from table1 with (nolock)
group by create_dt
) a
full outer join
(select create_dt,count( id) demo,row_number() over (order by create_dt) as row_num
from table2 with (nolock)
group by create_dt
) b
on a.row_num = b.row_num
full outer join
(select create_dt,count( id) bro,row_number() over (order by create_dt) as row_num
from table3 with (nolock)
group by create_dt
) c
on b.row_num = c.row_num
full outer join
(select create_dt,count(id) [set],row_number() over (order by create_dt) as row_num
from table4 with (nolock)
group by create_dt
) d
on c.row_num = d.row_num
full outer join
(select create_dt,count(id) vip,row_number() over (order by create_dt) as row_num
from table5 with (nolock)
group by create_dt
) e
on d.row_num = e.row_num
full outer join
(select create_dt,count(id) dev,row_number() over (order by create_dt) as row_num
from table6 with (nolock)
group by create_dt
) f
on e.row_num = f.row_num
full outer join
(select convert(varchar(8),create_ts,112) create_dt,count(id) conf,row_number() over (order by convert(varchar(8),create_ts,112)) as row_num
from table7 with (nolock)
group by convert(varchar(8),create_ts,112)
) g
on f.row_num = g.row_num
order by a.create_dt
select a.create_dt,con,bro,[set],conf,demo,vip,dev
from
(select create_dt,count( id) con,row_number() over (order by create_dt) as row_num
from table1 with (nolock)
group by create_dt
) a
full outer join
(select create_dt,count( id) demo,row_number() over (order by create_dt) as row_num
from table2 with (nolock)
group by create_dt
) b
on a.row_num = b.row_num
full outer join
(select create_dt,count( id) bro,row_number() over (order by create_dt) as row_num
from table3 with (nolock)
group by create_dt
) c
on b.row_num = c.row_num
full outer join
(select create_dt,count(id) [set],row_number() over (order by create_dt) as row_num
from table4 with (nolock)
group by create_dt
) d
on c.row_num = d.row_num
full outer join
(select create_dt,count(id) vip,row_number() over (order by create_dt) as row_num
from table5 with (nolock)
group by create_dt
) e
on d.row_num = e.row_num
full outer join
(select create_dt,count(id) dev,row_number() over (order by create_dt) as row_num
from table6 with (nolock)
group by create_dt
) f
on e.row_num = f.row_num
full outer join
(select convert(varchar(8),create_ts,112) create_dt,count(id) conf,row_number() over (order by convert(varchar(8),create_ts,112)) as row_num
from table7 with (nolock)
group by convert(varchar(8),create_ts,112)
) g
on f.row_num = g.row_num
order by a.create_dt
Wednesday, September 2, 2015
.NET 4.5 Framework Initialization Error
Today while trying to run an application on a server I got the below error.
By default Visual Studio 2012 uses .Net 4.5 for as the version for build and release of projects. Even though I changed the version to .Net 4.0 and rebuilt the application and copied to the server I still was getting the error.
The reason I was still getting this error was due to the runtime support still referring to .Net 4.5 framework in the App.config file.
After changing the runtime to .Net 4.0 the application ran without any error.
Other solution is to download .Net 4.5.
Friday, August 28, 2015
c# - Hashset vs List performance
In one of my recent tasks I had to work with text files and extract rows based on certain values contained in a specific column.
I had a 4 GB file and I had to parse that file to extract rows that matched certain keywords.The total keyword matches varied from 10000 to less than a million.
For a smaller set of keywords I have used List but noticed that as the list items grew in size the lookup operation became slower.Below is a test I did to compare the lookup performance between a List<string> and HashSet<string>
HashSet<string> lookup is faster and should be used for lookup instead of a list.
using System;
using System.Collections.Generic;
using System.Diagnostics;
namespace HashSetListCompare
{
class Program
{
static void Main(string[] args)
{
try
{
string s = "512367";
List<string> sNumbersList = new List<string>();
HashSet<string> sNumbersHashSet = new HashSet<string>();
//List load performance
Stopwatch oListLoad = new Stopwatch();
oListLoad.Start();
for (int i = 0; i < 1000000; i++)
sNumbersList.Add(i.ToString());
oListLoad.Stop();
Console.WriteLine("List load time:" + oListLoad.Elapsed);
//Hash Set load performance
Stopwatch oHashSetLoad = new Stopwatch();
oHashSetLoad.Start();
for (int i = 0; i < 1000000; i++)
sNumbersHashSet.Add(i.ToString());
oHashSetLoad.Stop();
Console.WriteLine("Hash load time:" + oHashSetLoad.Elapsed);
//List lookup performance
Stopwatch oListWatch = new Stopwatch();
oListWatch.Start();
if(sNumbersList.Contains(s))
Console.Write("List lookup time: ");
oListWatch.Stop();
Console.WriteLine(oListWatch.Elapsed);
//Hash set lookup performance
Stopwatch oHashSetWatch = new Stopwatch();
oHashSetWatch.Start();
if(sNumbersHashSet.Contains(s))
Console.Write("Hash lookup time: ");
oHashSetWatch.Stop();
Console.WriteLine(oHashSetWatch.Elapsed);
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
Console.WriteLine(ex.StackTrace);
}
Console.WriteLine("Done");
Console.Read();
}
}
}
Thursday, July 23, 2015
Hadoop - Decompress gz files from reducer output
Due to space constraints on our Hadoop clusters we had store output files in compressed gz format.
STORE finaldata INTO '/output/${month}/${folder}.gz/' USING PigStorage('\t');
Once we started storing our output files in the compressed format, we had to change the way we merge the output files to extract it to the local system.The getmerge command copied the files in compressed format.
hadoop fs -getmerge /output/${month}/${folder}.gz/part-* /output/handoff.txt
In order to decompress the files and copy it over to local system we had to use the text command..
hadoop fs -text /output/${month}/${folder}.gz/part-* > /output/handoff.txt
STORE finaldata INTO '/output/${month}/${folder}.gz/' USING PigStorage('\t');
Once we started storing our output files in the compressed format, we had to change the way we merge the output files to extract it to the local system.The getmerge command copied the files in compressed format.
hadoop fs -getmerge /output/${month}/${folder}.gz/part-* /output/handoff.txt
In order to decompress the files and copy it over to local system we had to use the text command..
hadoop fs -text /output/${month}/${folder}.gz/part-* > /output/handoff.txt
Friday, July 17, 2015
pig - Extract data from large Bags after GROUP BY
I have multiple files with same columns and I wanted to aggregate the values in two columns using SUM.
The column structure is below
ID first_count second_count name desc
1 10 10 A A_Desc
1 25 45 A A_Desc
1 30 25 A A_Desc
2 20 20 B B_Desc
2 40 10 B B_Desc
In wanted the below output
ID first_count second_count name desc
1 65 80 A A_Desc
2 60 30 B B_Desc
Below is the script I wrote .
A = LOAD '/output/*/part*' AS (id:chararray,first_count:int,second_count:int,name:chararray,desc:chararray);
B = GROUP A BY id;
C = FOREACH B GENERATE group as id,
SUM(A.first_count) as first_count,
SUM(A.second_count) as second_count,
A.name as name,
A.desc as desc;
This resulted in the the below output.The output has multiple tuples for each group i.e. the name and desc were repeated.
1 65 80 {(A)(A)(A)}{(A_Desc)(A_Desc)( A_Desc)}
2 60 30 {(B)(B)} {(B_Desc)( B_Desc)}
When I ran this script on the entire dataset that has 500 millions rows in it the reducer got stuck since the Bags after the group had large number of tuples.
In order to get the desired output.I had to distinct the tuple i.e. name and desc and then FLATTEN them to get the desired output.
D = FOREACH C
{
distinctnamebag = DISTINCT name;
distinctdescbag = DISTINCT desc;
GENERATE id,first_count,second_count,flatten(distinctnamebag) as name, flatten(distinctdescbag) as desc;
}
Now the output looks like this
1 65 80 A A_Desc
2 60 30 B B_Desc
The column structure is below
ID first_count second_count name desc
1 10 10 A A_Desc
1 25 45 A A_Desc
1 30 25 A A_Desc
2 20 20 B B_Desc
2 40 10 B B_Desc
In wanted the below output
ID first_count second_count name desc
1 65 80 A A_Desc
2 60 30 B B_Desc
Below is the script I wrote .
A = LOAD '/output/*/part*' AS (id:chararray,first_count:int,second_count:int,name:chararray,desc:chararray);
B = GROUP A BY id;
C = FOREACH B GENERATE group as id,
SUM(A.first_count) as first_count,
SUM(A.second_count) as second_count,
A.name as name,
A.desc as desc;
This resulted in the the below output.The output has multiple tuples for each group i.e. the name and desc were repeated.
1 65 80 {(A)(A)(A)}{(A_Desc)(A_Desc)( A_Desc)}
2 60 30 {(B)(B)} {(B_Desc)( B_Desc)}
When I ran this script on the entire dataset that has 500 millions rows in it the reducer got stuck since the Bags after the group had large number of tuples.
In order to get the desired output.I had to distinct the tuple i.e. name and desc and then FLATTEN them to get the desired output.
D = FOREACH C
{
distinctnamebag = DISTINCT name;
distinctdescbag = DISTINCT desc;
GENERATE id,first_count,second_count,flatten(distinctnamebag) as name, flatten(distinctdescbag) as desc;
}
Now the output looks like this
1 65 80 A A_Desc
2 60 30 B B_Desc
Wednesday, July 8, 2015
Increase process priority in Windows Server 2008
If you have used the task scheduler in windows server 2008 to schedule a windows task,you will see that by default the task scheduler runs the process with a Below Normal priority.
When I moved the tasks from windows server 2003 to windows server 2008, I noticed a sharp decline in performance.Most of the tasks that I have scheduled are multi threaded and IO intensive.
Even though the task settings is set to run under an Admin account with highest privileges the process runs with a Below Normal priority.
Note:You can change the priority here but I did not notice any change in the performance.
One way to bump up the process priority is in code
System.Diagnostics.Process.GetCurrentProcess().PriorityClass = ProcessPriorityClass.High
However I did not see any improvement in process performance.
After playing with the settings I was able to tweak the configuration file for the task in the task scheduler to set the process priority to High.The process now executes faster and I did see performance improvement over the previous process execution time.
Below are the screenshots of how to override windows server task scheduler process priority.
Create the task in the task scheduler.Once the task is created,right click on the task and choose export.This exports the task properties/settings to a xml file.Save the xml file on the local machine.Delete the task that was created.
Open the xml file which has the task settings and you will see the process priority set to 7.
Edit the priority and set it the desired priority.4 is for Normal and 1 is for High.Note that setting a higher priority is not recommended.My process is highly IO intensive and hence I have used 1.
Save the xml file.Go to the task scheduler,right click in the task list window and select import.Select the xml file for the process and the task should be created with a higher process priority.
Now my process runs with a High priority.Also the process performance has been higher. Even though the recommended priority is Normal, after 3 months of execution I have not encountered any issues with the process or the server.
When I moved the tasks from windows server 2003 to windows server 2008, I noticed a sharp decline in performance.Most of the tasks that I have scheduled are multi threaded and IO intensive.
Even though the task settings is set to run under an Admin account with highest privileges the process runs with a Below Normal priority.
Note:You can change the priority here but I did not notice any change in the performance.
One way to bump up the process priority is in code
System.Diagnostics.Process.GetCurrentProcess().PriorityClass = ProcessPriorityClass.High
However I did not see any improvement in process performance.
After playing with the settings I was able to tweak the configuration file for the task in the task scheduler to set the process priority to High.The process now executes faster and I did see performance improvement over the previous process execution time.
Below are the screenshots of how to override windows server task scheduler process priority.
Create the task in the task scheduler.Once the task is created,right click on the task and choose export.This exports the task properties/settings to a xml file.Save the xml file on the local machine.Delete the task that was created.
Open the xml file which has the task settings and you will see the process priority set to 7.
Edit the priority and set it the desired priority.4 is for Normal and 1 is for High.Note that setting a higher priority is not recommended.My process is highly IO intensive and hence I have used 1.
Save the xml file.Go to the task scheduler,right click in the task list window and select import.Select the xml file for the process and the task should be created with a higher process priority.
Now my process runs with a High priority.Also the process performance has been higher. Even though the recommended priority is Normal, after 3 months of execution I have not encountered any issues with the process or the server.
Wednesday, June 24, 2015
wpf - Application to convert string case
For the most part at my work place, programmers follow certain coding conventions.However,there are multiple teams that work on separate projects and there is no hard fast rule regarding coding standards.
Lower Case
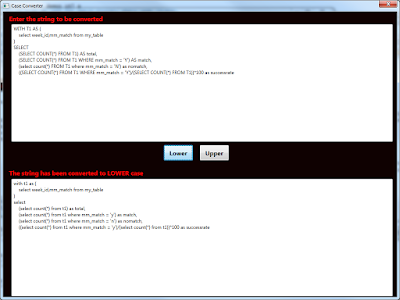
Upper case
I work for the platform team and my role involves converting and upgrading projects.When it comes to coding standards, I would definitely like to follow certain coding standards so that the code remains consistent and is easy to read.
Often, during upgrades,rewrites I end up with code that has case mixed up all over the place.Below is a very small piece of sql with mixed case.Usually I deal with SQL that is several hundred of lines and I find code with mixed case especially in sql server as an eye sore.Sorry for being a stickler :)
WITH T1 AS (
select * from my_table
)
SELECT
(SELECT COUNT(*) FROM T1) AS total,
(SELECT COUNT(*) FROM T1 WHERE mm_match = 'Y') AS match,
(select count(*) FROM T1 where mm_match = 'N') as nomatch,
((select count(*) FROM T1 WHERE mm_match = 'Y')/(select count(*) FROM T1))*100 as successrate
SQL server is case insensitive and ideally I like to keep the sql code case,table name,stored procedures names and the rest of the database object names in lower case.
I created a small WPF application top handle this.Below is the application that helps me in converting the case of the sql to lower or upper case.
The XAML is below
<Window x:Class="StringCaseConverter.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Case Converter" Height="768" Width="1024" ResizeMode="NoResize" Background="#FF0F0101">
<Grid>
<TextBox Name="UserStringTextBox" HorizontalAlignment="Left" Height="300" TextWrapping="Wrap" VerticalAlignment="Top" Width="975" Margin="22,32,0,0" AcceptsReturn="True"/>
<TextBox Name="ConvertedStringTextBox" HorizontalAlignment="Left" Height="300" TextWrapping="Wrap" VerticalAlignment="Top" Width="975" Margin="22,428,0,0" AcceptsReturn="True"/>
<Button Name="LowerButton" Content="Lower" HorizontalAlignment="Left" Margin="411,341,0,0" VerticalAlignment="Top" Width="75" RenderTransformOrigin="-0.329,0.32" Height="40" FontSize="16" FontWeight="Bold" Click="LowerButton_Click"/>
<Button Name="UpperButton" Content="Upper" HorizontalAlignment="Left" Margin="503,341,0,0" VerticalAlignment="Top" Width="75" Height="40" FontSize="16" FontWeight="Bold" Click="UpperButton_Click"/>
<Label Content="Enter the string to be converted" HorizontalAlignment="Left" Margin="10,2,0,0" VerticalAlignment="Top" Width="257" FontWeight="Bold" FontSize="16" Foreground="#FFF90808"/>
<Label Name="CaseLabel" Content="" HorizontalAlignment="Left" Margin="10,397,0,0" VerticalAlignment="Top" RenderTransformOrigin="-2.544,0.327" Width="402" FontWeight="Bold" FontSize="16" Foreground="#FFFD0404"/>
</Grid>
</Window>
The code
using System.Windows;
namespace StringCaseConverter
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void LowerButton_Click(object sender, RoutedEventArgs e)
{
string s = UserStringTextBox.Text.Trim();
if (string.IsNullOrEmpty(s))
MessageBox.Show("Empty String ! Enter a string to be converted.","Warning",MessageBoxButton.OK,MessageBoxImage.Warning);
else
{
ConvertedStringTextBox.Text = s.ToLower();
CaseLabel.Content = "The string has been converted to LOWER case";
}
}
private void UpperButton_Click(object sender, RoutedEventArgs e)
{
string s = UserStringTextBox.Text.Trim();
if (string.IsNullOrEmpty(s))
MessageBox.Show("Empty String ! Enter a string to be converted.","Warning",MessageBoxButton.OK,MessageBoxImage.Warning);
else
{
ConvertedStringTextBox.Text = s.ToUpper();
CaseLabel.Content = "The string has been converted to UPPER case";
}
}
}
}
Lower Case
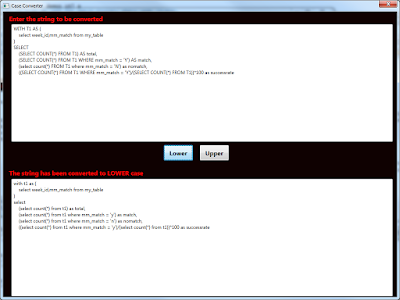
Upper case
sql - count total rows,subset of rows and percentage
Recently in one of the projects, I had to get the total number of rows along with count of a subset of rows and the percentage of the subset of rows compared to the total rows.
The table structure is very simple with just two columns Id,Match.The Id column is of type int and Match column takes two values 'Y' and 'N' indicating whether a match was found or not in the given Id.We use this table as a reporting table to record some activity.
For reporting purposes we wanted to get the total records sent for match in a given week and wanted to know the success rate.
Below is the query to get the total count,match count,nomatch count and the success rate.
select
id,count(*) total,
count(case when match='Y' then 1 end) match,
count(case when match='N' then 1 end) nomatch,
(cast(count(case when match='Y' then 1 end) as float)/cast(count(*) as float)) * 100 successrate
from
my_table
my_table
group by
id
id
order by
id desc
id desc
Monday, June 22, 2015
ASP pages on IIS - Active Server Pages Error 0221 Invalid @ Command Directive
Setting up asp pages that use asp.net code behind pages to handle the requests will require additional steps to be configured on IIS. I had to upgrade one of the sites which had few asp pages.I did not have the option to rewrite the page as aspx page and hence had to host it on the IIS server an asp page.
The site was upgraded to use 4.0 .Net engine and hosted on IIS 8.5. The aspx pages worked as expected,however I stared getting asp error 0221 when I tried to access the asp urls.
Below is the error screenshot.
The asp file has a page directive indicating that the language is c# and that it uses a code behind page aspx.cs to process the requests.
In order to get the asp page with asp.net code behind file to work, I had to indicate that the page will be processed by the asp.net engine. Below are the screenshots of the steps required to get the asp page to be processed by asp.net engine on IIS 8.5.
Open Inetmgr and navigate to Handler Mappings in the features section for the site hosting the asp page with asp.net code behind page.
Double Click the Handler Mappings features to bring up all the extension mappings.
Double Click ASPClassic to edit the handler.You will notice that the ASPClassic pages are handled by the asp.dll. This makes the asp page not recognize the page directives since the directives are for the asp.net engine.
Click the ellipses and navigate to the appropriate .Net framework directory and choose the aspnet_isapi.dll as the handler.In my case the site has been built in .Net 4.0 version.
Note:For applications built on/for 64-Bit servers the aspnet_isapi.dll handler should be from Framework64 folder.
Once the asp.net handler has been mapped.Restart IIS and navigate to the asp page and the error should be resolved.Note that when you do the above handler mapping changes, the configuration file for you site will be updated with the new settings.
<system.webServer>
<handlers>
<remove name="ASPClassic" />
<add name="ASPClassic" path="*.asp" verb="GET,HEAD,POST" modules="IsapiModule" scriptProcessor="C:\Windows\Microsoft.NET\Framework\v4.0.30319\aspnet_isapi.dll" resourceType="File" requireAccess="Script" preCondition="bitness32" />
</handlers>
</system.webServer>
The page no long throws the asp error and returns a xml tag as it supposed in my application.
Hope that helps !
The site was upgraded to use 4.0 .Net engine and hosted on IIS 8.5. The aspx pages worked as expected,however I stared getting asp error 0221 when I tried to access the asp urls.
Below is the error screenshot.
The asp file has a page directive indicating that the language is c# and that it uses a code behind page aspx.cs to process the requests.
In order to get the asp page with asp.net code behind file to work, I had to indicate that the page will be processed by the asp.net engine. Below are the screenshots of the steps required to get the asp page to be processed by asp.net engine on IIS 8.5.
Open Inetmgr and navigate to Handler Mappings in the features section for the site hosting the asp page with asp.net code behind page.
Double Click the Handler Mappings features to bring up all the extension mappings.
Double Click ASPClassic to edit the handler.You will notice that the ASPClassic pages are handled by the asp.dll. This makes the asp page not recognize the page directives since the directives are for the asp.net engine.
Click the ellipses and navigate to the appropriate .Net framework directory and choose the aspnet_isapi.dll as the handler.In my case the site has been built in .Net 4.0 version.
Note:For applications built on/for 64-Bit servers the aspnet_isapi.dll handler should be from Framework64 folder.
Once the asp.net handler has been mapped.Restart IIS and navigate to the asp page and the error should be resolved.Note that when you do the above handler mapping changes, the configuration file for you site will be updated with the new settings.
<system.webServer>
<handlers>
<remove name="ASPClassic" />
<add name="ASPClassic" path="*.asp" verb="GET,HEAD,POST" modules="IsapiModule" scriptProcessor="C:\Windows\Microsoft.NET\Framework\v4.0.30319\aspnet_isapi.dll" resourceType="File" requireAccess="Script" preCondition="bitness32" />
</handlers>
</system.webServer>
The page no long throws the asp error and returns a xml tag as it supposed in my application.
Hope that helps !
Subscribe to:
Posts (Atom)