For the most part at my work place, programmers follow certain coding conventions.However,there are multiple teams that work on separate projects and there is no hard fast rule regarding coding standards.
Lower Case
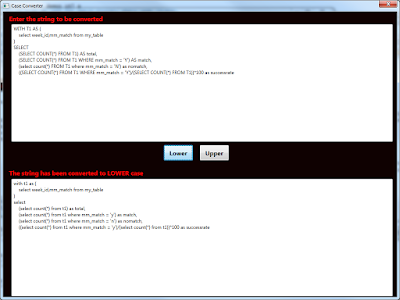
Upper case
I work for the platform team and my role involves converting and upgrading projects.When it comes to coding standards, I would definitely like to follow certain coding standards so that the code remains consistent and is easy to read.
Often, during upgrades,rewrites I end up with code that has case mixed up all over the place.Below is a very small piece of sql with mixed case.Usually I deal with SQL that is several hundred of lines and I find code with mixed case especially in sql server as an eye sore.Sorry for being a stickler :)
WITH T1 AS (
select * from my_table
)
SELECT
(SELECT COUNT(*) FROM T1) AS total,
(SELECT COUNT(*) FROM T1 WHERE mm_match = 'Y') AS match,
(select count(*) FROM T1 where mm_match = 'N') as nomatch,
((select count(*) FROM T1 WHERE mm_match = 'Y')/(select count(*) FROM T1))*100 as successrate
SQL server is case insensitive and ideally I like to keep the sql code case,table name,stored procedures names and the rest of the database object names in lower case.
I created a small WPF application top handle this.Below is the application that helps me in converting the case of the sql to lower or upper case.
The XAML is below
<Window x:Class="StringCaseConverter.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Case Converter" Height="768" Width="1024" ResizeMode="NoResize" Background="#FF0F0101">
<Grid>
<TextBox Name="UserStringTextBox" HorizontalAlignment="Left" Height="300" TextWrapping="Wrap" VerticalAlignment="Top" Width="975" Margin="22,32,0,0" AcceptsReturn="True"/>
<TextBox Name="ConvertedStringTextBox" HorizontalAlignment="Left" Height="300" TextWrapping="Wrap" VerticalAlignment="Top" Width="975" Margin="22,428,0,0" AcceptsReturn="True"/>
<Button Name="LowerButton" Content="Lower" HorizontalAlignment="Left" Margin="411,341,0,0" VerticalAlignment="Top" Width="75" RenderTransformOrigin="-0.329,0.32" Height="40" FontSize="16" FontWeight="Bold" Click="LowerButton_Click"/>
<Button Name="UpperButton" Content="Upper" HorizontalAlignment="Left" Margin="503,341,0,0" VerticalAlignment="Top" Width="75" Height="40" FontSize="16" FontWeight="Bold" Click="UpperButton_Click"/>
<Label Content="Enter the string to be converted" HorizontalAlignment="Left" Margin="10,2,0,0" VerticalAlignment="Top" Width="257" FontWeight="Bold" FontSize="16" Foreground="#FFF90808"/>
<Label Name="CaseLabel" Content="" HorizontalAlignment="Left" Margin="10,397,0,0" VerticalAlignment="Top" RenderTransformOrigin="-2.544,0.327" Width="402" FontWeight="Bold" FontSize="16" Foreground="#FFFD0404"/>
</Grid>
</Window>
The code
using System.Windows;
namespace StringCaseConverter
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void LowerButton_Click(object sender, RoutedEventArgs e)
{
string s = UserStringTextBox.Text.Trim();
if (string.IsNullOrEmpty(s))
MessageBox.Show("Empty String ! Enter a string to be converted.","Warning",MessageBoxButton.OK,MessageBoxImage.Warning);
else
{
ConvertedStringTextBox.Text = s.ToLower();
CaseLabel.Content = "The string has been converted to LOWER case";
}
}
private void UpperButton_Click(object sender, RoutedEventArgs e)
{
string s = UserStringTextBox.Text.Trim();
if (string.IsNullOrEmpty(s))
MessageBox.Show("Empty String ! Enter a string to be converted.","Warning",MessageBoxButton.OK,MessageBoxImage.Warning);
else
{
ConvertedStringTextBox.Text = s.ToUpper();
CaseLabel.Content = "The string has been converted to UPPER case";
}
}
}
}
Lower Case
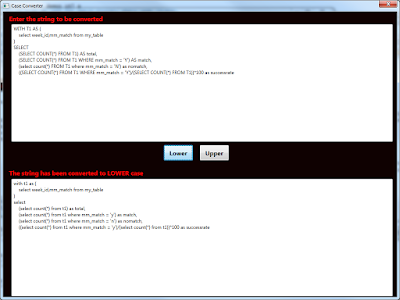
Upper case