In this post I will go through the steps needed to create a Windows Communcation Foundation service,client. The example is very basic and will illustrate the minimum steps required to create a WCF application.
The steps to create a WCF application are
Generate the proxy using svcutil
Start your client and enter the seed,range values.Your service host should print the Seed and Range sent from the client and generate the random number and send it back to the client.
The steps to create a WCF application are
- Define a WCF service contract
- Implement a WCF service contract
- Host/Run the WCF service
- Create a WCF client
- Configure the WCF client
- Consume the WCF service using WCF client
Step 1: Design the WCF service contract
To start with create a console application in Visual Studio 2010 and choose new project -> console application.Give it a name
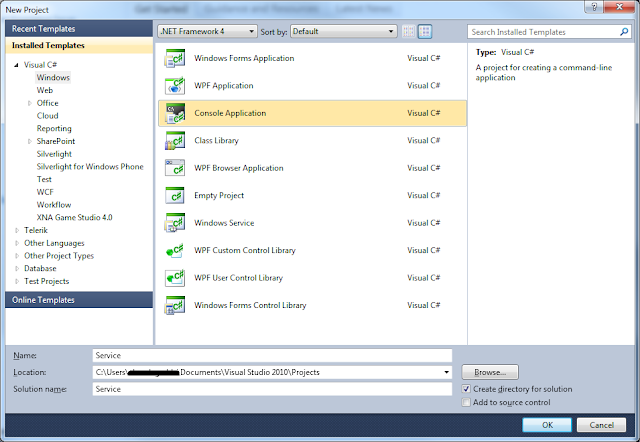
Add a reference System.ServiceModel
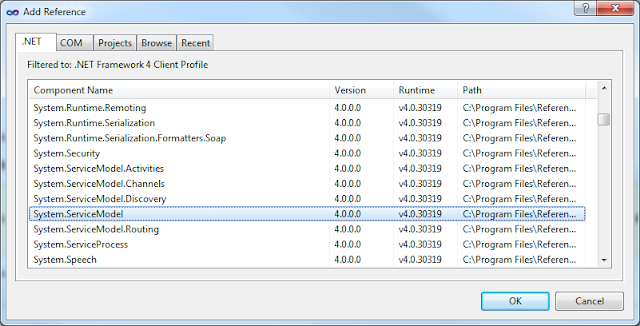
Define a ServiceContract in the program.cs by creating an Interface and define a method as an OperationContract
[ServiceContract(Namespace = "http://RandomNumberService")]
public interface IRandomNumberGenerator
{
[OperationContract]
int GetRandomNumber(int iSeed, int iRange);
}
Program.cs would now look like this
using System;
using System.ServiceModel;
namespace RandomNumberService
{
[ServiceContract(Namespace = "http://RandomNumberService")]
public interface IRandomNumberGenerator
{
[OperationContract]
int GetRandomNumber(int iSeed, int iRange);
}
class Program
{
static void Main(string[] args)
{
}
}
}
Step 2: Implement the WCF Service Contract.
Add a class to program.cs file and implement the interface defined in Step 1
class RandomNumberGenerator : IRandomNumberGenerator
{
public int GetRandomNumber(int p_iSeed, int p_iRange)
{
Console.WriteLine("Seed: " + p_iSeed.ToString() + " Range: " + p_iRange.ToString());
Random oRandom = new Random();
int iRandomNumber = oRandom.Next(p_iSeed,p_iRange);
Console.WriteLine("Random Number generated: " + iRandomNumber.ToString());
return iRandomNumber;
}
}
Program.cs would now look like this
using System;
using System.ServiceModel;
namespace RandomNumberService
{
[ServiceContract(Namespace = "http://RandomNumberService")]
public interface IRandomNumberGenerator
{
[OperationContract]
int GetRandomNumber(int iSeed, int iRange);
}
class Program
{
static void Main(string[] args)
{
}
}
class RandomNumberGenerator : IRandomNumberGenerator
{
public int GetRandomNumber(int p_iSeed, int p_iRange)
{
Console.WriteLine("Seed: " + p_iSeed.ToString() + " Range: " + p_iRange.ToString());
Random oRandom = new Random();
int iRandomNumber = oRandom.Next(p_iSeed,p_iRange);
Console.WriteLine("Random Number generated: " + iRandomNumber.ToString());
return iRandomNumber;
}
}
}
Step 3:Host and run the service.
Inorder to host the service, you need to create a base address,service host at the base address,configure the service host's endpoint and start the service host.
The above steps are listed in the below code
//Create a base address for your service host
Uri oBaseAddress = new Uri("http://localhost:12345/ServiceExample/Service");
//Create a service host using the base address
ServiceHost oServiceHost = new ServiceHost(typeof(RandomNumberGenerator), oBaseAddress);
try
{
ServiceHost.AddServiceEndpoint(typeof(IRandomNumberGenerator), new WSHttpBinding(), "RandomNumberGenerator");
ServiceMetadataBehavior oServiceMetadataBehavior = new ServiceMetadataBehavior();
oServiceMetadataBehavior.HttpGetEnabled = true;
oServiceHost.Description.Behaviors.Add(oServiceMetadataBehavior);
oServiceHost.Open();
Console.WriteLine("The service is started.");
Console.WriteLine("Press any key to stop service.")
Console.WriteLine();
Console.ReadLine();
oServiceHost.Close();
}
catch (CommunicationException ex)
{
Console.WriteLine(ex.Message);
oServiceHost.Abort();
}
Step 4: Create the WCF Client
In the solution explorer create new project --> console application.Give it a name
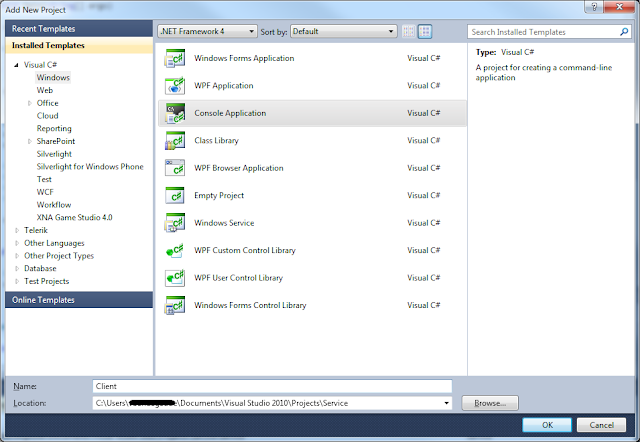
Add System.ServiceModel refernce to your client
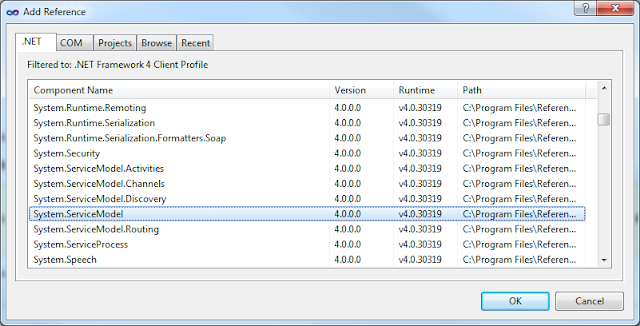
Next step is to generate the proxy using the svcutil.exe
Using the Visual Studio Command Prompt go to C:Windows\System32 and use the scvutil.exe to generate the proxy.
Make sure the service is started before you try to generate the proxy class.
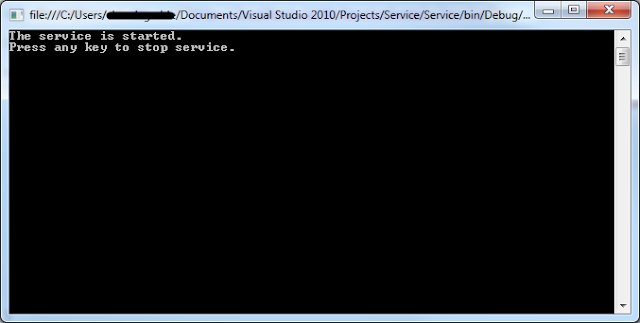
Generate the proxy using svcutil
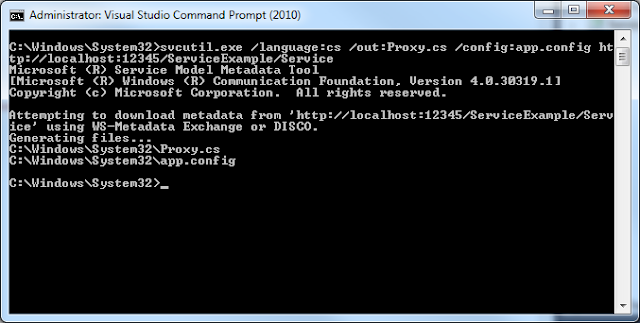
Next import the proxy.cs and app.config into your client project
The proxy.cs has the below auto generated code
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated by a tool.
// Runtime Version:4.0.30319.269
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
[System.CodeDom.Compiler.GeneratedCodeAttribute("System.ServiceModel", "4.0.0.0")]
[System.ServiceModel.ServiceContractAttribute(Namespace="http://RandomNumberService", ConfigurationName="IRandomNumberGenerator")]
public interface IRandomNumberGenerator
{
[System.ServiceModel.OperationContractAttribute(Action="http://RandomNumberService/IRandomNumberGenerator/GetRandomNumber", ReplyAction="http://RandomNumberService/IRandomNumberGenerator/GetRandomNumberResponse")]
int GetRandomNumber(int iSeed, int iRange);
}
[System.CodeDom.Compiler.GeneratedCodeAttribute("System.ServiceModel", "4.0.0.0")]
public interface IRandomNumberGeneratorChannel : IRandomNumberGenerator, System.ServiceModel.IClientChannel
{
}
[System.Diagnostics.DebuggerStepThroughAttribute()]
[System.CodeDom.Compiler.GeneratedCodeAttribute("System.ServiceModel", "4.0.0.0")]
public partial class RandomNumberGeneratorClient : System.ServiceModel.ClientBase<IRandomNumberGenerator>, IRandomNumberGenerator
{
public RandomNumberGeneratorClient()
{
}
public RandomNumberGeneratorClient(string endpointConfigurationName) :
base(endpointConfigurationName)
{
}
public RandomNumberGeneratorClient(string endpointConfigurationName, string remoteAddress) :
base(endpointConfigurationName, remoteAddress)
{
}
public RandomNumberGeneratorClient(string endpointConfigurationName, System.ServiceModel.EndpointAddress remoteAddress) :
base(endpointConfigurationName, remoteAddress)
{
}
public RandomNumberGeneratorClient(System.ServiceModel.Channels.Binding binding, System.ServiceModel.EndpointAddress remoteAddress) :
base(binding, remoteAddress)
{
}
public int GetRandomNumber(int iSeed, int iRange)
{
return base.Channel.GetRandomNumber(iSeed, iRange);
}
}
Step 5: Configure the WCF Client.
Import the app.config to the client and you should see the endpoint configured.
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<system.serviceModel>
<bindings>
<wsHttpBinding>
<binding name="WSHttpBinding_IRandomNumberGenerator" closeTimeout="00:01:00"
openTimeout="00:01:00" receiveTimeout="00:10:00" sendTimeout="00:01:00"
bypassProxyOnLocal="false" transactionFlow="false" hostNameComparisonMode="StrongWildcard"
maxBufferPoolSize="524288" maxReceivedMessageSize="65536"
messageEncoding="Text" textEncoding="utf-8" useDefaultWebProxy="true"
allowCookies="false">
<readerQuotas maxDepth="32" maxStringContentLength="8192" maxArrayLength="16384"
maxBytesPerRead="4096" maxNameTableCharCount="16384" />
<reliableSession ordered="true" inactivityTimeout="00:10:00"
enabled="false" />
<security mode="Message">
<transport clientCredentialType="Windows" proxyCredentialType="None"
realm="" />
<message clientCredentialType="Windows" negotiateServiceCredential="true"
algorithmSuite="Default" />
</security>
</binding>
</wsHttpBinding>
</bindings>
<client>
<endpoint address="http://localhost:12345/ServiceExample/Service/RandomNumberGenerator"
binding="wsHttpBinding" bindingConfiguration="WSHttpBinding_IRandomNumberGenerator"
contract="IRandomNumberGenerator" name="WSHttpBinding_IRandomNumberGenerator">
<identity>
<userPrincipalName value="myname@office.mycompany.com" />
</identity>
</endpoint>
</client>
</system.serviceModel>
</configuration>
Step 6:
Consume the WCF service using WCF client
In the progam.cs main method file of the client project write the code to consume the methods from the hosted service
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.ServiceModel;
namespace Client
{
class Program
{
static void Main(string[] args)
{
RandomNumberGeneratorClient oClient = new RandomNumberGeneratorClient();
Console.WriteLine("Please enter the seed for random number generator");
int iSeed = int.Parse(Console.ReadLine());
Console.WriteLine("Please enter the range for the random number generator");
int iRange = int.Parse(Console.ReadLine());
Console.WriteLine("Random Number returned from the service: " + oClient.GetRandomNumber(iSeed, iRange));
oClient.Close();
Console.WriteLine("Press any key to stop the client");
Console.Read();
}
}
}
Compile the client code and now you are all set to run your WCF application
Output:
Run the host first
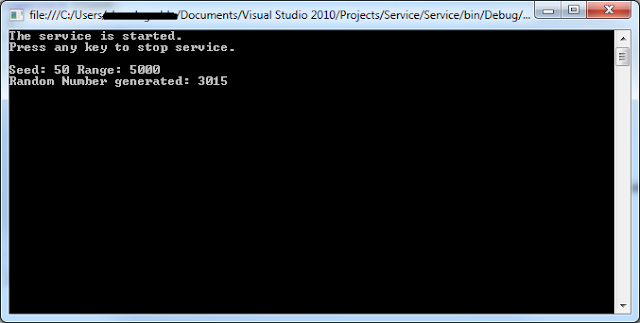
Start your client and enter the seed,range values.Your service host should print the Seed and Range sent from the client and generate the random number and send it back to the client.
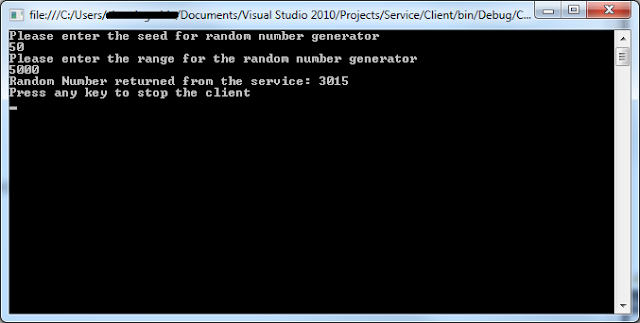
That's it, There you have mine,your first WCF application.FUN !!!
No comments:
Post a Comment